Have you considered how top platforms are built? Hashnode is powered by the MERN stack, while giants like Facebook and Netflix utilize React and Node.js for dynamic frontends and robust backends.
🔍 What is MERN Stack?
Ever wondered how you can build dynamic web applications with ease? The MERN stack is one of the go-to choices for developers, combining simplicity with power. This full-stack JavaScript framework enables developers to create smooth, interactive web experiences from the frontend to the backend. Whether you’re a beginner or an experienced developer, the MERN stack helps you deliver highly scalable, dynamic applications.
But what makes the MERN stack so special? Let’s dive into its components and see how it all comes together.
Components of the MERN Stack
The MERN stack is a powerful web development framework that comprises four key components:
M - MongoDB
E - Express.js
R - ReactJs
N - Node.js
Together, these tools create a robust ecosystem for full-stack development, from storing data to rendering beautiful UIs.
MongoDB
Ever struggled with rigid database schemas? MongoDB provides flexibility that traditional databases lack. It stores data in a document-oriented format, which means you can evolve your data structure as your application grows.
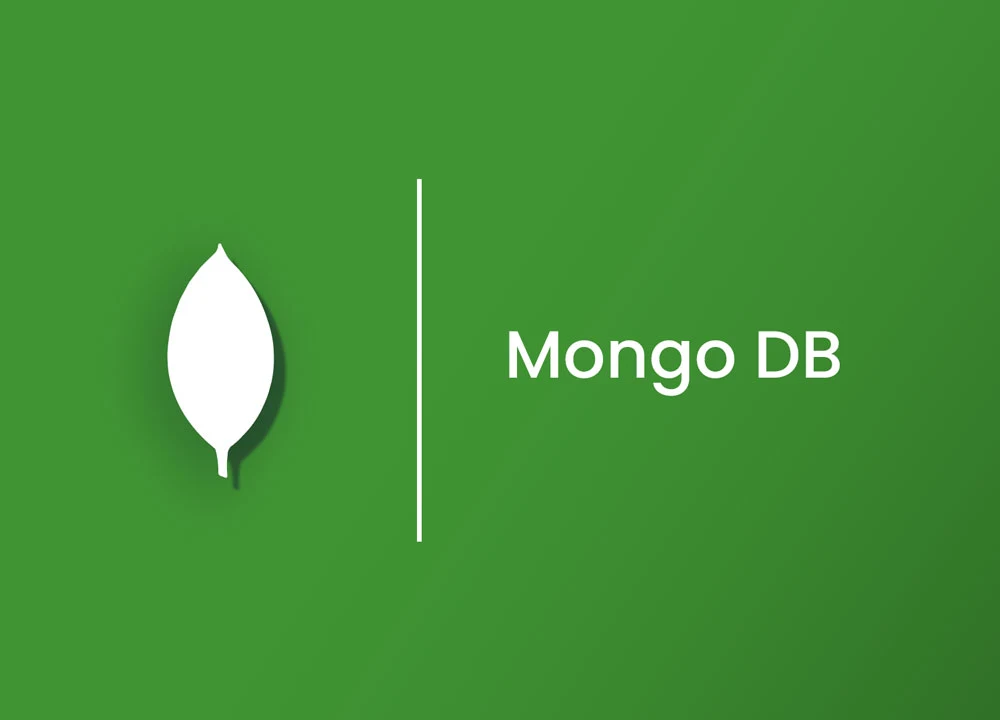
MongoDB
MongoDB is a NoSQL, document-oriented database designed to handle flexible, schema-less data structures, providing a modern alternative to traditional relational databases.
Key Features of MongoDB
Document-Oriented: Stores data in JSON-like documents.
Schema-less: Allows dynamic fields and evolving structures.
Powerful Query Capabilities: Supports rich query operations, including geospatial and aggregation.
Indexing: Efficient indexing to speed up queries.
Scalability and Performance: Scales horizontally through sharding, distributing data across servers, and supports replication for high availability.
Real-world example: Imagine building an online store where you need to add new product categories over time—MongoDB makes this process seamless without the need to redesign the entire database!
Express.js
Ever wondered how platforms like Uber or Twitter handle millions of requests every day? Express.js is the secret sauce behind many fast, scalable web applications. It makes building APIs and handling server-side logic a breeze.
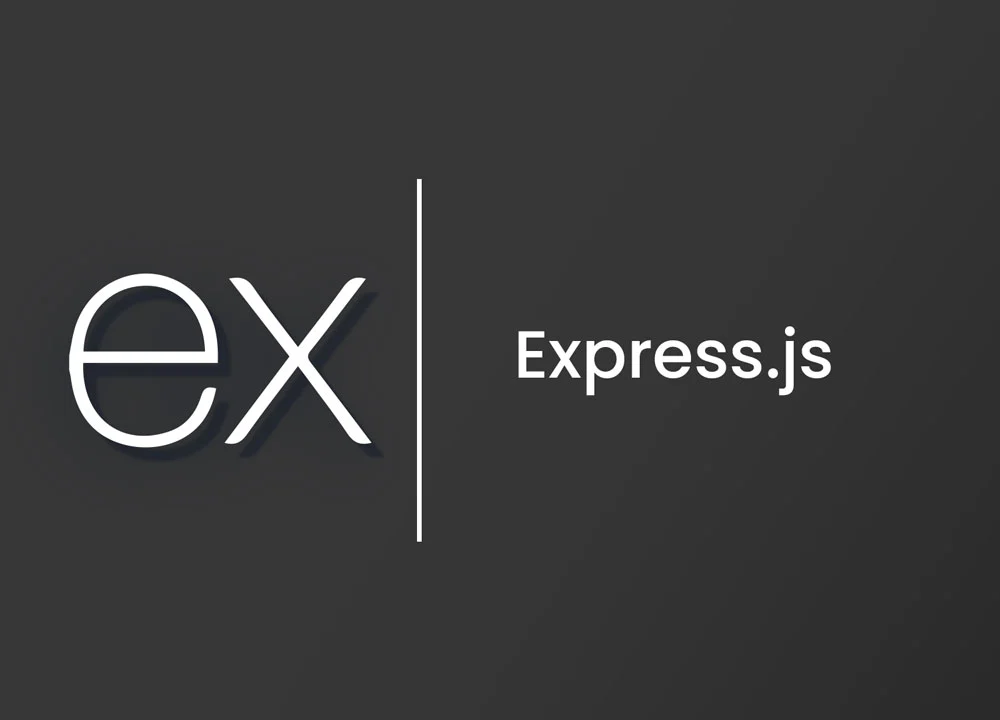
Express.js
Express.js is a free, open-source web application framework for Node.js, designed for rapid and efficient web application development. It simplifies the creation of APIs and web applications using JavaScript, enabling developers to build multi-page, single-page, or hybrid applications with ease.
Key Features of Express.js
Faster Server-Side Development: Enhances development speed and efficiency.
Middleware: Facilitates access to databases and client requests.
Routing: Provides advanced routing capabilities for URL management.
Debugging: Features a powerful debugging engine for easier bug removal.
Templating: Supports templating engines for dynamic HTML generation.
Advantages of Express.js
Ease of Learning: Offers a simpler learning curve for developers.
Frontend and Backend Integration: Works seamlessly with Node.js for comprehensive solutions.
Performance: Utilizes Node.js's V8 engine for rapid execution of JavaScript code.
With Express.js, your server becomes a highly efficient tool that can handle multiple client requests in real-time, making your web apps both responsive and scalable.
ReactJS
Want to build interactive UIs without the headache? React makes it simple to create dynamic web interfaces that users love. Whether you're building a single-page app or adding complex UI components, React has you covered.
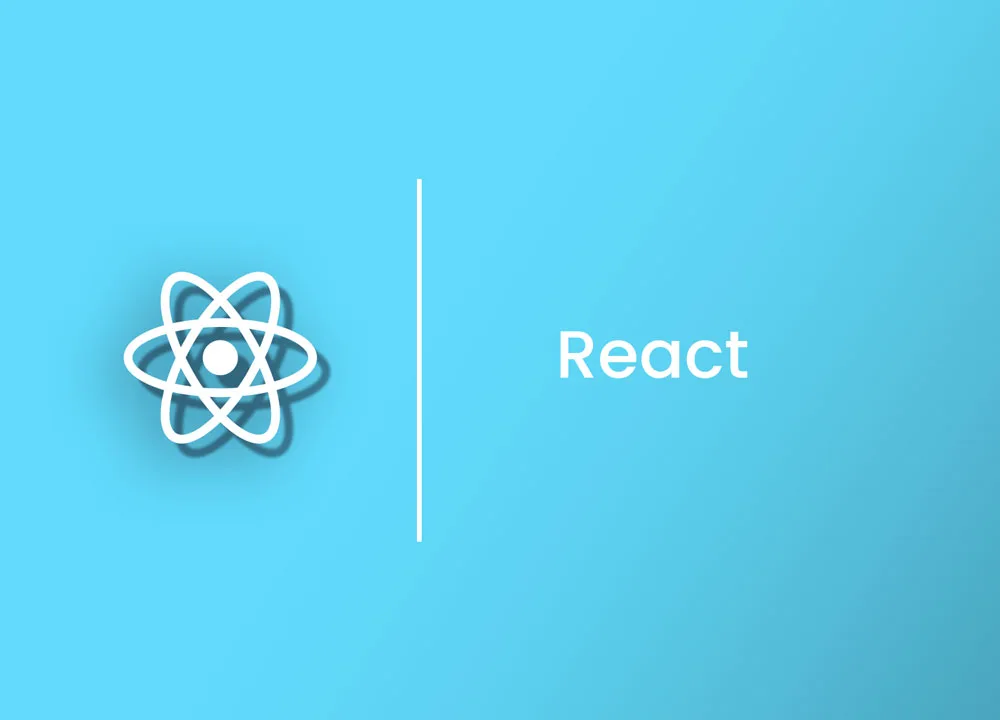
ReactJS
ReactJS has become a cornerstone of modern web development. It is a JavaScript library developed by Facebook for building dynamic user interfaces. Here’s a short view at ReactJS, a go-to choice for developers.
Key Features of ReactJS
Components
JSX (JavaScript XML)
Virtual DOM
State Management
State Management Solution
Props (Properties)
React Router
Hooks
Components
React lets you create components, reusable UI elements for your app. Components which are self-contained, reusable building blocks for your user interface. By breaking down the UI into manageable parts, React makes it easy to develop and maintain applications.
Example
/* Navbar.jsx */
function Navbar(props) {
return <nav> Navbar... </nav>;
}
/* App.jsx */
function App() {
return (
<Navbar />
<h1>Home Page</h1>
)
}
The App
component renders the Navbar
component followed by the rest of the code. This demonstrates how components are reused and composed in React.
JSX (JavaScript XML)
JSX lets you use HTML-like syntax in JavaScript files, improving readability. This markup is converted into standard JavaScript, facilitating a seamless blend of UI elements and logic.
Example
const element = <h1>Hello, world!</h1>;
function Greeting() {
return (
<div>
{element}
<p>Welcome to our website!</p>
</div>
);
}
In this example, JSX is used to define a heading element <h1>
and a Greeting
component. We can use that element
in this file wherever we want.
Virtual DOM
One of React’s key innovations is its Virtual DOM. React updates the Virtual DOM first, which then compares with the real DOM to determine the most efficient way to update the UI. This process enhances performance, especially in applications with complex interfaces.
State Management
React Hooks, functional components manage state effortlessly, facilitating dynamic updates to the user interface and improving overall interactivity.
Example
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
The Counter
component uses the useState
hook to manage the count
state. Each time the button is clicked, setCount(count + 1)
is called increasing the count
value, and the UI updates automatically.
State Management Solutions
Libraries in React like Redux and the Context API provide centralized state management solutions, allowing for efficient data sharing across multiple components, thus simplifying the state management process. It could be used to manage the state in applications especially when tracking is required for user inputs or AI responses.
Props (Properties)
Props are used to pass data from parent components to child components. It allows components to receive and render varying information based on their context.
Example
/* Greeting.jsx */
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
/* App.jsx */
function App() {
return <Greeting name="Alice" />;
}
The Greeting
component uses props
to display a customized greeting message. The App
component (parent of Greeting
component) passes the name
prop with the value "Alice" to the Greeting
component, which renders "Hello, Alice!". This demonstrates how data can be passed between components using props.
React Router
For single-page applications (SPAs), React Router is an essential library that enables seamless navigation between different views without requiring full page reloads.
Example
function App() {
return (
<Router>
<Switch>
<Route path="/about" component={About} />
<Route path="/" component={Home} />
</Switch>
</Router>
);
}
The App
component uses React Router to define routes for different views (Home
and About
). The Switch
component ensures that only one route is rendered at a time, based on the URL path.
Hooks
Introduced in React 16.8, hooks enable functional components to access state, and lifecycle features previously exclusive to class components. Common hooks like useState
for state management and useEffect
for side effects enhance React's functional programming capabilities.
Example
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const timer = setInterval(() => setSeconds(seconds => seconds + 1), 1000);
return () => clearInterval(timer);
}, []);
return <h1>Seconds: {seconds}</h1>;
}
The Timer
component uses the useState
hook to track the number of seconds and the useEffect
hook to set up an interval that increments the seconds every second. This demonstrates how hooks are used for state and side-effect management.
Why Choose React?
Dynamic Application Development: React simplifies dynamic web application creation, reducing code complexity while maintaining robust functionality.
Performance Optimization: The Virtual DOM in React boosts performance by minimizing direct manipulation of the real DOM.
Reusable Components: React's component-based architecture promotes reusability, cutting down development time and enhancing maintainability.
Unidirectional Data Flow: Its unidirectional data flow makes debugging easier by providing a clear trace of data origins and flow.
Easy Learning Curve: React blends HTML and JavaScript, making it easy for developers to learn and adopt.
Versatile for Web and Mobile Apps: With React Native, developers can build both web and mobile applications using the same framework.
Dedicated Debugging Tools: React offers dedicated debugging tools like the React Developer Tools extension, streamlining the debugging process.
Why Developers Love React: React’s component-based architecture allows you to reuse code, making your development process faster. And with React Hooks, you can manage state in your app without switching to class-based components. It’s like having a toolkit for building modern, dynamic applications without any clutter.
Node.js
What if you could use JavaScript to build both the frontend and backend of your application? With Node.js, that’s exactly what you can do. Node transforms JavaScript into a full-stack language, so you only need to master one language to build the entire application.
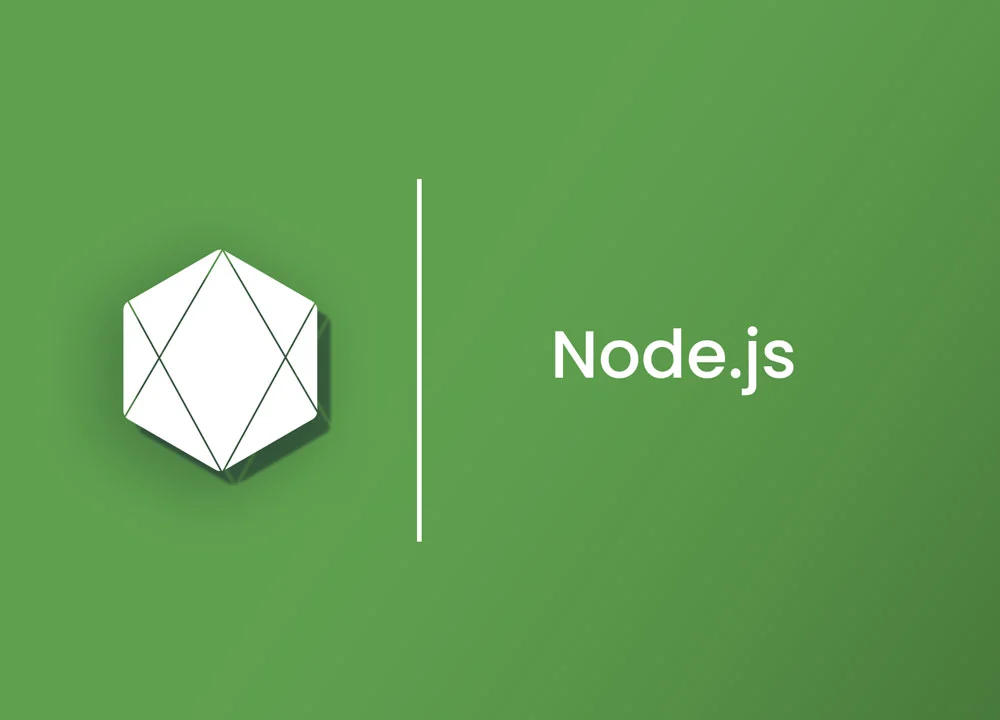
Node.js
Node.js is a runtime environment that allows developers to run JavaScript on the server side. Built on Google Chrome’s V8 JavaScript engine. The advent of Node.js transformed JavaScript into a full-stack language, capable of running both on the client and the server.
Key Features of Node.js
Event-Driven Architecture: Node.js uses an event-driven, non-blocking I/O model (a system that allows multiple tasks to happen at once without waiting), handling multiple tasks simultaneously asynchronously.
Non-Blocking I/O: Its asynchronous I/O allows Node.js to process multiple requests concurrently.
Single-Threaded Model: Node.js runs on a single thread but manages many connections at once through its non-blocking architecture.
NPM (Node Package Manager): NPM provides a vast library of open-source modules, simplifying many tasks.
Why Use Node.js?
JavaScript Everywhere: Enables consistent code across server-side and client-side, streamlining development with a unified language.
Rich Ecosystem: Access to over 2.1 million npm packages accelerates development, providing tools for various functionalities.
Real-Time Capabilities: Excellent for apps requiring real-time updates, like online gaming or messaging, using event-driven libraries like Socket.io.
Microservices & API Development: Ideal for building scalable microservices and APIs due to its lightweight runtime.
Active Community: Large, supportive developer community, offering abundant resources for learning and support.
Node.js is ideal for applications that need to process data in real-time, like online games, chat apps, or streaming services. Companies like LinkedIn and PayPal have used Node.js to increase the performance of their applications.
🚀 Boosting Applications with MERN Stack & AI
As the digital world evolves, combining MERN with artificial intelligence (AI) can create highly dynamic and intelligent applications. Imagine building a chatbot that talks to users or integrating image recognition in your web app!
Chatbots: Utilize Dialogflow or Microsoft Bot Framework to create intelligent chatbots that handle user interactions and store data in MongoDB.
Chat Interfaces: Tools or libraries like React Chatbot Kit, Microsoft Chat UI and Material UI are designed for building customizable chatbot interfaces in React.
Natural Language Processing (NLP): Integrate libraries like Natural or APIs such as Google Cloud Natural Language directly into your Node.js backend for dynamic text processing.
Recommendation Systems: Leverage machine learning models in your Node.js server to analyze user interactions stored in MongoDB for personalized suggestions.
Image and Video Analysis: Integrate APIs like Google Vision or Amazon Rekognition to seamlessly analyze user-generated content within your application.
Voice Recognition: Implement voice command features using libraries like annyang or APIs like Google Speech-to-Text, enabling interactive user experiences.
Sentiment Analysis: Employ AI tools in Node.js to analyze user feedback and comments in real-time, directly influencing app behavior.
Predictive Analytics: Build and integrate machine learning models that predict user behavior based on real-time data stored in MongoDB.
Real-time Data Processing: Run models in the browser using TensorFlow.js for real-time predictions without sending data back to the server.
Automated Testing: Use AI-driven testing tools like Testim to automate tests, adapting to changes in the application without extensive manual input.
User Personalization: Analyze user data stored in MongoDB to deliver highly personalized experiences based on real-time interactions.
Real-Time Communication: Real-time chat functionality can be added by integrating libraries like socket.io with backend (Node.js) and Frontend (React).
As we’ve covered the basics of the MERN stack, now it’s time to bring it all together. In our next blog, we’ll be building our first full-stack app step-by-step combining the MERN technology, so you can see how they work in harmony.
Ready to supercharge your development skills? Subscribe now and never miss the latest tips, tricks, and tools to master the MERN stack!
Let’s Discuss📌 Let’s Wrap It Up
The MERN stack is a powerhouse for building dynamic and efficient web applications. By mastering MongoDB, Express.js, React, and Node.js, you’ll have the skills to create full-stack apps that are fast, scalable, and future-ready. So, what are you waiting for? Jump into the MERN world and unlock your potential!
🌟 Subscribe now and start your MERN journey today!